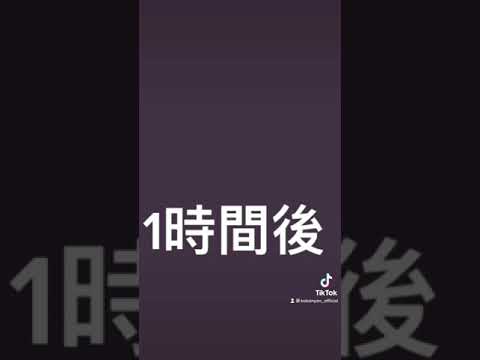
swift-youtube player 在 コバにゃんチャンネル Youtube 的精選貼文
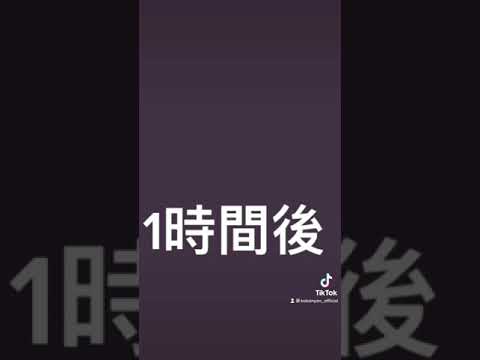
Severity: Notice
Message: Trying to get property 'plaintext' of non-object
Filename: models/Crawler_model.php
Line Number: 228
Backtrace:
File: /var/www/html/KOL/voice/application/models/Crawler_model.php
Line: 228
Function: _error_handler
File: /var/www/html/KOL/voice/application/controllers/Pages.php
Line: 336
Function: get_dev_google_article
File: /var/www/html/KOL/voice/public/index.php
Line: 319
Function: require_once
Severity: Notice
Message: Trying to get property 'plaintext' of non-object
Filename: models/Crawler_model.php
Line Number: 229
Backtrace:
File: /var/www/html/KOL/voice/application/models/Crawler_model.php
Line: 229
Function: _error_handler
File: /var/www/html/KOL/voice/application/controllers/Pages.php
Line: 336
Function: get_dev_google_article
File: /var/www/html/KOL/voice/public/index.php
Line: 319
Function: require_once
Search
Swift library for embedding and controlling YouTube videos in your iOS applications via WKWebView! - GitHub - gilesvangruisen/Swift-YouTube-Player: Swift ... ... <看更多>
The youtube-ios-player-helper
is an open source library that helps you embed a
YouTube iframe player into an iOS application. The library creates a
WebView
and a bridge between your application’s Objective-C code and the
YouTube player’s JavaScript code, thereby allowing the iOS application to control the
YouTube player. This article describes the steps to install the library and get started
using it from your iOS application.
This article assumes you have created a new Single View Application iOS project targeting
the latest version of iOS, and that you add the following files when creating the
project:
Main.storyboard
ViewController.h
ViewController.m
You can install the library via
CocoaPods or by copying the library
and source files from the
project’s GitHub page.
If your project uses CocoaPods, add the line below to your Podfile to install the library.
In that line, replace x.y.z
with the latest pod version, which will be
identified on the project’s GitHub page.
pod "youtube-ios-player-helper", "~> x.y.z"
At the command line prompt, type pod install
to update your workspace with the
dependencies.
Tip: Remember that when using CocoaPods, you must open the .xcworkspace
file
in Xcode, not the .xcodeproj
file.
Check out the CocoaPods
tutorial to learn more.
To install the helper library manually, either download the source via
GitHub’s download link or
clone the repository. Once you have a local copy of the code, follow these steps:
Open the sample project in Xcode or Finder.
Select YTPlayerView.h
, YTPlayerView.m
, and the
Assets folder. If you open the workspace in Xcode, these are available
under Pods -> Development Pods -> YouTube-Player-iOS-Helper and
Pods -> Development Pods -> YouTube-Player-iOS-Helper -> Resources. In the Finder,
these are available in the project's root directory in the Classes and
Assets directories.
Drag these files and folders into your project. Make sure the Copy items into
destination group’s folder option is checked. When dragging the Assets folder, make
sure that the Create Folder References for any added folders option is
checked.
The library should now be installed.
YTPlayerView
via Interface Builder or the Storyboard To add a YTPlayerView
via Interface Builder or the Storyboard:
Drag a UIView
instance onto your View.
Select the Identity Inspector and change the class of the view to
YTPlayerView
.
Open ViewController.h
and add the following header:
#import “YTPlayerView.h”
Also add the following property:
@property(nonatomic, strong) IBOutlet YTPlayerView *playerView;
In Interface Builder, create a connection from the View element that you defined in the
previous step to your View Controller's playerView
property.
Now open ViewController.m
and add the following code to the end of your
viewDidLoad
method:
[self.playerView loadWithVideoId:@"M7lc1UVf-VE"];
Build and run your application. When the video thumbnail loads, tap the video thumbnail to
launch the fullscreen video player.
The ViewController::loadWithVideoId:
method has a variant,
loadWithVideoId:playerVars:
, that allows developers to pass additional player
variables to the view. These player variables correspond to the
player parameters in the
IFrame Player API. The playsinline
parameter enables the video to play
directly in the view rather than playing fullscreen. When a video is playing inline, the
containing iOS application can programmatically control playback.
Replace the loadWithVideoId:
call with this code:
NSDictionary *playerVars = @{
@"playsinline" : @1,
};
[self.playerView loadWithVideoId:@"M7lc1UVf-VE" playerVars:playerVars];
Open up the storyboard or Interface Builder. Drag two buttons onto your View, labeling them
Play and Stop. Open ViewController.h
and add these methods, which
will be mapped to the buttons:
- (IBAction)playVideo:(id)sender;
- (IBAction)stopVideo:(id)sender;
Now open ViewController.m
and define these two functions:
- (IBAction)playVideo:(id)sender {
[self.playerView playVideo];
}- (IBAction)stopVideo:(id)sender {
[self.playerView stopVideo];
}
Most of the IFrame Player API functions have Objective-C equivalents, though some of the
naming may differ slightly to more closely match Objective-C coding guidelines. Notable
exceptions are methods controlling the volume of the video, since these are controlled by
the phone hardware or with built in UIView
instances designed for this purpose,
such as MPVolumeView
.
Open your storyboard or Interface Builder and control-drag to connect the Play and
Stop buttons to the playVideo:
and stopVideo:
methods.
Now build and run the application. Once the video thumbnail loads, you should be able to
play and stop the video using native controls in addition to the player controls.
It can be useful to programmatically handle playback events, such as playback state changes
and playback errors. In the JavaScript API, this is done with
event listeners.
In Objective-C,this is done with a
delegate.
The following code shows how to update the interface declaration in
ViewController.h
so the class conforms to the delegate protocol. Change
ViewController.h
’s interface declaration as follows:
@interface ViewController : UIViewController<YTPlayerViewDelegate>
YTPlayerViewDelegate
is a protocol for handling playback events in the player.
To update ViewController.m
to handle some of the events, you first need to set
the ViewController
instance as the delegate of the YTPlayerView
instance. To make this change, add the following line to the viewDidLoad
method
in ViewController.h
.
self.playerView.delegate = self;
Now add the following method to ViewController.m
:
- (void)playerView:(YTPlayerView *)playerView didChangeToState:(YTPlayerState)state {
switch (state) {
case kYTPlayerStatePlaying:
NSLog(@"Started playback");
break;
case kYTPlayerStatePaused:
NSLog(@"Paused playback");
break;
default:
break;
}
}
Build and run the application. Watch the log output in Xcode as the player state changes.
You should see updates when the video is played or stopped.
The library provides the constants that begin with the kYT*
prefix for
convenience and readability. For a full list of these constants, look at
YTPlayerView.m
.
The library builds on top of the IFrame Player API by creating a WebView
and
rendering the HTML and JavaScript required for a basic player. The library's goal is to be
as easy-to-use as possible, bundling methods that developers frequently have to write into a
package. There are a few limitations that should be noted:
YTPlayerView
instances. If your application has multipleYTPlayerView
instances, a recommended best practice is to pause or stopNSNotificationCenter
to dispatch notifications that playback is about toYTPlayerView
instances. Reuse your existing, loaded YTPlayerView
instances when possible. When aUIView
instance or aYTPlayerView
instance, and don't call either loadVideoId:
orloadPlaylistId:
. Instead, use thecueVideoById:startSeconds:
family of functions, which do notWebView
. There is a noticeable delay when loading the entireSince this is an open-source project, please submit fixes and improvements to the
master branch of the GitHub
project.
#1. gilesvangruisen/Swift-YouTube-Player - GitHub
Swift library for embedding and controlling YouTube videos in your iOS applications via WKWebView! - GitHub - gilesvangruisen/Swift-YouTube-Player: Swift ...
#2. Embed YouTube Videos in iOS Applications with the YouTube ...
The youtube-ios-player-helper is an open source library that helps you embed a YouTube iframe player into an iOS application.
#4. How to embed YouTube videos into your iOS app using Swift
In this example, we are going to add the YouTube Player iOS Helper library into our project using Cocaopods. ... Next, in the swift file of your ...
#5. iOS開發(swift) youtube的後台播放問題 - iT 邦幫忙
youtube. ios. swift. api. 後台播放. vvv236. 2 年前‧ 1170 瀏覽. 檢舉. 0. 各位大大好目前我在用YouTube-Player-iOS-Helper開發一個音樂APP, 我設定了後台播放,
#6. Swift-YouTube-Player : Not able to read available videos
To understand what happened to your streaming, you should add another parameter, so following the Swift-YouTube-Player example correct this ...
#7. NicholasMata/YoutubePlayer as Swift Package - Swiftpack.co
NicholasMata/YoutubePlayer as Swift Package - A port of youtube-ios-player-helper written in Swift with SPM support.
#8. Swift-YouTube-Player Alternatives - iOS Video | LibHunt
Swift -YouTube-Player alternatives and similar libraries · ZFPlayer. 9.7 3.7 L3 Swift-YouTube-Player VS ZFPlayer · LFLiveKit. 9.4 0.0 L2 Swift- ...
#9. youtube-ios-player-helper-swift - A full swift implementation of ...
The only official way of playing a YouTube video inside an app is with a web view and the iframe player API. Unfortunately, this is very slow and quite ugly, so ...
#10. Swift-YouTube-Player:无法阅读可用的视频 - IT工具网
原文 标签 swift youtube youtube-data-api youtube-javascript-api. 我正在使用https://github.com/gilesvangruisen/Swift-YouTube-Player在我的应用中读取youtube ...
#11. YouTube player for SwiftUI | swiftobc
This component is distributed as a Swift package. Just add this repo's URL to XCode: https://github.com/globulus/swiftui-youtube-player ...
#12. How to play YouTube video in iOS with swift 5 - Weps Tech
And also I will tell what are the techniques you need to keep in mind while you are working for the YouTube video player.
#13. SwiftUI YouTube Player for iOS and MacOS - iOS Example
This component is distributed as a Swift package. Just add this repo's URL to XCode: https://github.com/globulus/swiftui-youtube-player ...
#14. Can we get normal quality of YouTube video using Safari ...
Or good quality is only possible with official YouTube app on iOS? ... https://github.com/gilesvangruisen/Swift-YouTube-Player. YouTube IFrame API methods ...
#15. 关于swift:具有YouTube v3 API和youtube-ios-player-helper的 ...
我在使用Google / YouTube提供的 youtube-ios-player-helper 窗格自动播放视频时遇到问题。 这是我的应用程序的相关部分(iOS 10,Swift 3):.
#16. Integrating YouTube into Your iOS Applications - CODE ...
Run YouTube Videos in iOS Applications. ... native video player component for playing back YouTube content from within your application.
#17. swift youtube player,大家都在找解答 旅遊日本住宿評價
swift youtube player ,大家都在找解答第1頁。The youtube-ios-player-helper is an open source library that helps you embed a YouTube iframe player into an iOS ...
#18. youtube-ios-player-helper-swift - Cocoa Controls
youtube -ios-player-helper-swift. Apache 2.0 License. Swift. iOS. Favorite ... to add YouTube video playback in their applications via the iframe player in a ...
#19. How To Embed YouTube Videos in an iOS App with Swift 4
It seems as though the helper library youtube-ios-player-helper does ... embed YouTube videos into any iOS App using Swift 4 and Xcode 10.
#20. YoutubeKit is a video player that fully supports Youtube ...
YoutubeKit. YoutubeKit is a video player that fully supports Youtube IFrame API and YoutubeDataAPI to easily create Youtube applications. Swift ...
#21. 如何在iOS Swift中自動播放YouTube視訊(無需使用者互動)
【IOS】如何在iOS Swift中自動播放YouTube視訊(無需使用者互動) ... firstScriptTag); var player; function onYouTubePlayerAPIReady() { player = new YT.
#22. To play Youtube Video in iOS app | Newbedev
YouTube has functionality to load any video. ... Or this one: https://github.com/gilesvangruisen/Swift-YouTube-Player.
#23. Using YTPlayerView to play embedded YouTube video in iOS ...
Watch on YouTube". Note that there is no such issue when playing some other videos. Is there any way to address the above issue? I could use iframe to ...
#24. Play a video – SwiftUI – Hacking with Swift forums
import AVKit import SwiftUI struct ContentView: View { var body: some View { VideoPlayer(player: AVPlayer(url: URL(string: "https://www.youtube.
#25. How to Play Video with Animation using AVPlayer (Ep 16)
#26. Getting elapsed time for YouTube videos on iOS - Fresh ...
Then fortunately my colleague found a great link to Youtube Player API reference where we could query for current video time periodically by ...
#27. Swift / youtube api - libs.garden
Swift library for embedding and controlling YouTube videos in your iOS ... YoutubeKit is a video player that fully supports Youtube IFrame API and ...
#28. [Swift] YouTube-Player-iOS-Helper を使って YouTube 動画を ...
はじめに 先日、クラスメソッド関連会社の underscore よりマイクラTubeという、YouTube上にあるマインクラフト動画を再生するアプリをリリースしま ...
#29. Enable Airplay? - Swift-YouTube-Player | GitAnswer
Swift -YouTube-Player ... mediaPlaybackAllowsAirPlay = true" to the swift file manually but that does not work. Any ideas? Thanks!
#30. YouTube Player 사용하기 - 뀔뀔(swieeft)의 개발새발기
YouTube Player 사용하기. #iOS #Swift. swieeft | 2020. 04. 09. 13:40. 안녕하세요. 오랜만에 블로그에 글을 남깁니다. 오늘은 유튜브 플레이어 사용 방법에 대해 ...
#31. Watch a police officer admit to playing Taylor Swift ... - The Verge
“You can record all you want,” he said, according to a video obtained by The Verge. “I just know it can't be posted to YouTube.” ...
#32. 封裝WKWebView播放YouTuBe視頻的播放器 - 程式前沿
ps:上個項目做過有關YouTube視頻播放的功能,之前在GitHub上搜的是 ... 創建一個< iframe >(和YouTube播放器)zPUdFID3lmo M7lc1UVf-VE var player; ...
#33. youtube_player_flutter | Flutter Package
Flutter plugin for playing or streaming inline YouTube videos using the official iFrame player API. ... iOS: --ios-language swift , Xcode version >= 11 ...
#34. Play a video from Youtube in a AVPlayerViewController in Swift
Play a video from Youtube in a AVPlayerViewController in Swift ... NSURL(string: "https://youtu.be/d88APYIGkjk") let player = AVPlayer(URL: videoURL!) let ...
#35. swift youtube player experience(Others-Community) - TitanWolf
swift youtube player experience. 1: youtube player can play video by id or url. The two main methods used are loadWithVideoId and loadVideoByURL.
#36. Swift-YouTube-Playe, a library for embedding ... - Reddit
Swift -YouTube-Playe, a library for embedding, playing, controlling YouTube videos in your iOS apps. r/swift - Swift-YouTube-Playe, a library for embedding, ...
#37. youtube-ios-player-helper-swift 上的UIWebView 到WKWebView
我的应用程序使用框架youtube-is-player-helper-swift,在这里找到:ht.
#38. YouTubePlayer.swift - Adding YouTube Video to Swift IPhone
VideoPlayerView.swift // YouTubePlayer // // Created by Giles Van Gruisen on 12/21/14. // Copyright (c) 2014 Giles Van Gruisen. All rights reserved.
#39. swift - Autoplay YouTube videos in WKWebView with iOS 11
I want to have small YouTube player in my app. The only way i found to be ... another YouTube player url?
#40. [iOS/Swift] Youtube Player 만들기 (썸네일 불러오기, 영상 재생 ...
[iOS/Swift] Youtube Player 만들기 (썸네일 불러오기, 영상 재생하기). choidam 2020. 5. 1. 03:23. 간단한 유튜브 재생기를 만들어보자. Video Class
#41. How to Make the Use of YouTube Player in Swift? - AegisISC ...
So, you can play and see youtube videos in the iOS application by using Youtube Player API in Swift. IOS developers India have explained the ...
#42. Swift: YouTube - How to Display Video Player Controls (Ep 17)
#43. Youtube player in swift - Webapp Codes
Youtube player in swift. ... Following pod file is use for youtube player. pod 'youtube-ios-player-helper', '~> 0.1.6' ...
#44. Video Streaming Tutorial for iOS: Getting Started
Your goal is to show a video player whenever a user taps one of the cells. ... To get started, navigate to VideoFeedView.swift and add the ...
#45. iOSアプリにYoutubeプレイヤーを組み込む - Qiita
iOSアプリにYoutubeプレイヤーを組み込む. iOSCocoaPodsYouTubeSwiftplayer. アプリにYoutubeプレイヤーを組み込んで制御できます。 IMG_1516.
#46. Embedded Youtube not autoplaying video in UIWebView
ios - Center an embedded youtube video in a UIWebview Swift - Stack Ov.. ... let width: CGFloat = player.frame.size.width let height = ceil((width / 16) ...
#47. Swift youtube api - Category: Nem
There's also the Swift Youtube Quickstart for your reference. ... that helps you embed a YouTube iframe player into an iOS application.
#48. youtube-ios-player-helper-swift 1.0.3 on CocoaPods
Helper library for iOS developers looking to add YouTube video playback in their applications via the iframe player in a UIWebView.
#49. 使用YouTube API 打造影音搜尋App - 完整Swift 教學
YouTube API 非常容易使用,不過有些事情你最好先知道一下,否則使用起來可能會感覺卡卡的。在這篇Swift 教學,我們將會完成一支可以用來下載頻道和影片( Video ) ...
#50. Swift-YouTube-Player: не может читать доступные видео
Я использую https://github.com/gilesvangruisen/Swift-YouTube-Player чтобы читать видео YouTube в моем приложении, например это: ...
#51. Swift-YouTube-Player : не в состоянии читать доступные ...
Чтобы понять, что произошло с потоковой передачей, вам следует добавить еще один параметр, поэтому, следуя примеру Swift-YouTube-Player ...
#52. Swift Youtube Framework委托方法未触发 - 小空笔记
我正在使用一个框架在Swift中播放Youtube视频: https : github.com gilesvangruisen Swift YouTube Player 。 该框架提供了委托方法,但我似乎无法使 ...
#53. Build your own YOUTUBE Replica with Swift! | Udemy
Create the same feature you see in the YOUTUBE application. ... IOS 10, Xcode 8, Swift 3. ... Add and configure the AV Player in swift ...
#54. Getting YouTube embedded in IOS 9 using Swift | roaet
These are just basic notes for me because I will forget. This uses the Swift-YouTube-Player Cocoapod from ...
#55. Detect When, Iframe(Avplayer) Of Video Player Inside Wkwebkit
Swift over Coffee is a podcast that helps you keep your Swift skills up to ... YouTube Player iOS Helper is the official way to embed a youtube You can set ...
#56. YouTube-Player-iOS-Helper无法使用YTPlayerView类 - 码农 ...
您的Swift文件: import UIKit import youtube_ios_player_helper // You're missing this line class MyView: UIView { // Some class @IBOutlet var ...
#57. TAYLOR SWIFT: YouTube Presents Interview (English Subtitles)
#58. Play Youtube Videos in Background Using Youtube iOS ...
... not using swift and don't want to use dynamic frameworks use_frameworks! # pods for yourprojectname endnow add the line pod "youtube-ios-player-helper" ...
#59. YouTubePlayer-Swift - try {} except | Software developer's ...
404: Not Found. Latest podspec. { "name": "YouTubePlayer-Swift", "version": "1.0", "summary": "A swift port of the YouTube helper (embedded ...
#60. Cop Plays Taylor Swift Song to Block BLM Protest Video From ...
I just know it can't be posted to YouTube.” Later, the officer, identified as Sgt. David Shelby, reiterates to Burch, “I'm playing my music so ...
#61. 在Swift中使用YouTube-Player-iOS-Helper時找不到 ...
我遇到了與帖子YouTube-Player-iOS-Helper can't use YTPlayerView class中相同的問題。 但是,當我在後面的回答中發現https://stackoverflow.com/a/30719229在橋接頭 ...
#62. youtube player delegate in swift
youtube player delegate in swift. import UIKit ... install pods pod "youtube-ios-player-helper", "~> 0.1.4". override func viewDidLoad() {.
#63. youtube-ios-player-helper-swift 上的UIWebView 到WKWebView
我的应用程序使用框架youtube is player helper swift,在这里找到: https : github.com malkouz youtube ios player helper swift它是原始youtube ...
#64. IINA - The modern media player for macOS
Powered by the open source media player mpv, IINA can play almost every media file ... Online streams. YouTube playlists. Download browser extensions. Swift ...
#65. Vevo - Wikipedia
Vevo is an American multinational video hosting service, best known for providing music videos to YouTube. ... On August 28, 2017, both YouTube and Vevo records were eclipsed by Swift ...
#66. Taylor Swift to face trial in Shake It Off copyright case - BBC
Taylor Swift must face a jury trial over accusations that she copied ... Both tracks feature variations of the phrases "players gonna play" ...
#67. Floating for YouTube™
Always on top Floating Mini Player for YouTube™. App review: http://www.ilovefreesoftware.com/05/windows/internet/plugins/chrome-extension-watch ...
#68. YouTube Music 2021 Recap: how to relive your year in music
Here's what you need to know about YouTube's Spotify Unwrapped rival, ... from high school played the new Taylor Swift album this year.
#69. Taylor Swift to face plagiarism trial over Shake It Off lyrics
Both songs feature variations on the lines “players gonna play” and “haters gonna hate”. Reached by the Guardian, representatives for Swift ...
#70. Is D'Andre Swift playing today vs. the Broncos? Latest news ...
Dealing with a shoulder injury, is Detroit Lions running back D'Andre Swift playing Week 14 against the Denver Broncos?
#71. Taylor Swift fails in bid to shake off lyrics lawsuit - RTE
Singer Taylor Swift must face a lawsuit from songwriters who claim ... In Shake It Off, Swift sings: "the players gonna play, play, play, ...
#72. Taylor Swift to face plagiarism trial over Shake It Off lyrics - Metro
Meanwhile, in Playas Gon' Play – written by Sean Hall and Nathan Butler – 3LW sing the phrases: 'Players, they gonna play, and haters, they ...
#73. Taylor Swift must face copyright lawsuit over hit single Shake It ...
Hall and Butler said the combination of playas or players with hatas or haters was unique to its use in their song. The pair are seeking ...
#74. D'Andre Swift ruled OUT for Lions' Week 14 game against the ...
American football player and coach. Detroit Lions head coach Dan Campbell ended any potential drama with the status of running back D'Andre ...
#75. Jw player app - Itasat Rastreamento Veicular
JW FLV Player was the video player chosen and used by YouTube back when they ... Version 4 is a complete rewrite of the JW Player SDK for iOS in Swift, ...
#76. Report: Lions running back D'Andre Swift may land on IR after ...
As the Detroit Lions come off a week dealing with a flu outbreak, the resulting lengthy injury report and a run of players going on the COVID-19 ...
#77. NFL Week 14 injuries: Chargers' Keenan Allen, Lions' D'Andre ...
Receiver Noah Brown was the only other player listed on the Cowboys' ... may be without two of their top playmakers in Swift and Hockenson.
#78. Taylor Swift must face jury trial over Shake It Off copyright ... - ITV
Both songs feature the phrases "players gonna play" and "haters gonna hate". Judge Fitzgerald had previously ruled in 2018 the lyrics were too " ...
#79. Taylor Swift to face trial in 'Shake It Off' copyright court case
Taylor Swift will face a jury trial over accusations that she ... the chorus of Swift's 2014 single, in which she sings, “'Cause the players ...
#80. FedEx Players Air and Ground players of the week | NFL.com
Vote for the FedEx Ground Player of Week 14. Dalvin Cook ... Can't-Miss Play: D'Andre Swift's 57-yard TD run is his longest of career so far.
#81. NOTEBOOK: Hockenson doubtful, Swift ruled out for Lions ...
Between Hockenson and Swift combined, the Lions are missing out on ... Campbell said there were only three or four players expected to sit ...
#82. 愛瘋一切為蘋果的彼得潘iOS Swift App 程式設計- iOS上開發 ...
iOS上開發YouTube相關App十分容易,只要告訴UIWebView影片網址,即可舒舒服服地欣賞影片 ... 對影片做進階的操作,千萬別錯過Google官方推出的youtube-ios-player-helper !
#83. D'Andre Swift (shoulder) considered day-to-day, team 'hopeful ...
This is the same shoulder injury that occurred in Week 12's Thanksgiving game vs the Chicago Bears that has caused Swift to miss the last ...
#84. Who is the Detroit Lions' Player to Watch vs. Broncos?
Penei Sewell, Julian Okwara, the rookie DTs (Levi Onwuzurike and Alim McNeill) and D'Andre Swift come to mind. Okwara and Swift are already ...
#85. Watch: These are the top YouTube videos of 2021 - KXLG
#86. Acer United States | Laptops, Desktops, Chromebooks ...
next@acer. Global Press Conference 2021 made for humanity · ConceptD 7 Ezel · Predator Triton 500 · Swift X · PREDATOR TRITON 500SE · Windows 11 · Xbox Game Pass.
#87. Taylor Swift is celebrating her 32nd birthday - Audacy
Taylor Swift is celebrating her 32nd birthday. The All Too Well Singer turns 32 today and her career started in a very unique way at just 12 ...
#88. Inside Taylor Swift's Birthday Party – She's Feelin' 32! - Capital ...
Taylor Swift partied with the likes of Haim and Tommy Dorfman to celebrate turning 32 years old!
#89. See Taylor Swift's 32nd Birthday Party Photos - ELLE
Taylor Swift is celebrating her 32nd birthday today, and the singer opted to have a joint birthday party with Alana Haim, who turns 30 on ...
#90. British comedian Russell Brand questions Australia's ...
Session ID: 2021-12-14:515578f5eb59fe25bd7533ed Player Element ID: ... In an 11-minute video posted to his YouTube channel over the weekend, ...
#91. Lions players away from building to open week; under normal ...
ALLEN PARK -- The Detroit Lions had seven players land on the COVID ... D'Andre Swift (shoulder), T.J. Hockenson (foot) and Alex Anzalone ...
#92. Swift an IR candidate | Shark Bites
Swift an IR candidate | Shark Bites | Draft Sharks. ... »View all D'Andre Swift Shark Bites ». Related Players: Jamaal Williams. Buffalo ...
#93. Can't shake this: Taylor Swift to face copyright lawsuit
Pop superstar Taylor Swift must face a lawsuit from songwriters who claim ... In "Shake It Off," Swift sings: "the players gonna play, play, ...
#94. Taylor Swift Songs, Ranked: Her 10 Best (And 10 Worst)
If The Killers and Aloe Blacc can sing about being 'The Man', then why can't Taylor? The line “And they would toast to me, oh / Let the players ...
#95. Mokiii player from El Dorado abusing Speed exploits on ...
[CLICK VIDEO LINK BELOW TO YOUTUBE VIDEO]. O w O Esports Mokiii speed cheater - YouTube. What are the steps to reproduce the issue as you ...
#96. [Updated] Here's Apple iOS 15/iPadOS 15 update tracker
Swift Playgrounds to build and run apps directly on iPad to learn ... The iOS 15 video player now has YouTube-like video playback speed ...
#97. John Swift update emerges ahead of Reading's clash with Luton
Reading midfielder John Swift could potentially be available for next ... Quiz: What club did Reading FC sign each of these 29 players from?
swift-youtube player 在 gilesvangruisen/Swift-YouTube-Player - GitHub 的推薦與評價
Swift library for embedding and controlling YouTube videos in your iOS applications via WKWebView! - GitHub - gilesvangruisen/Swift-YouTube-Player: Swift ... ... <看更多>