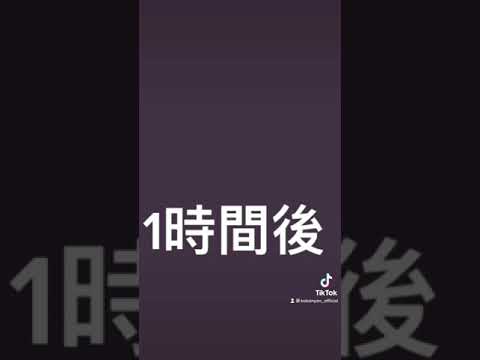
youtube-ios-player-helper 在 コバにゃんチャンネル Youtube 的最讚貼文
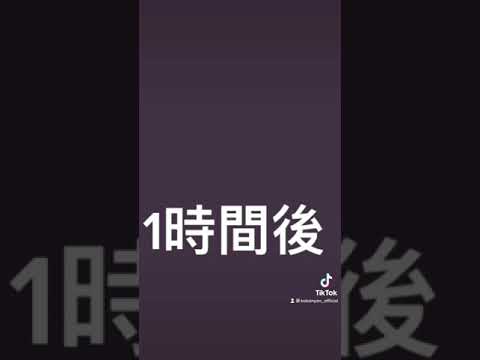
Severity: Notice
Message: Trying to get property 'plaintext' of non-object
Filename: models/Crawler_model.php
Line Number: 228
Backtrace:
File: /var/www/html/KOL/voice/application/models/Crawler_model.php
Line: 228
Function: _error_handler
File: /var/www/html/KOL/voice/application/controllers/Pages.php
Line: 336
Function: get_dev_google_article
File: /var/www/html/KOL/voice/public/index.php
Line: 319
Function: require_once
Severity: Notice
Message: Trying to get property 'plaintext' of non-object
Filename: models/Crawler_model.php
Line Number: 229
Backtrace:
File: /var/www/html/KOL/voice/application/models/Crawler_model.php
Line: 229
Function: _error_handler
File: /var/www/html/KOL/voice/application/controllers/Pages.php
Line: 336
Function: get_dev_google_article
File: /var/www/html/KOL/voice/public/index.php
Line: 319
Function: require_once
Search
Lightweight helper library that allows iOS developers to add inline playback of YouTube videos through a WebView - GitHub ... ... <看更多>
The youtube-ios-player-helper
is an open source library that helps you embed a
YouTube iframe player into an iOS application. The library creates a
WebView
and a bridge between your application’s Objective-C code and the
YouTube player’s JavaScript code, thereby allowing the iOS application to control the
YouTube player. This article describes the steps to install the library and get started
using it from your iOS application.
This article assumes you have created a new Single View Application iOS project targeting
the latest version of iOS, and that you add the following files when creating the
project:
Main.storyboard
ViewController.h
ViewController.m
You can install the library via
CocoaPods or by copying the library
and source files from the
project’s GitHub page.
If your project uses CocoaPods, add the line below to your Podfile to install the library.
In that line, replace x.y.z
with the latest pod version, which will be
identified on the project’s GitHub page.
pod "youtube-ios-player-helper", "~> x.y.z"
At the command line prompt, type pod install
to update your workspace with the
dependencies.
Tip: Remember that when using CocoaPods, you must open the .xcworkspace
file
in Xcode, not the .xcodeproj
file.
Check out the CocoaPods
tutorial to learn more.
To install the helper library manually, either download the source via
GitHub’s download link or
clone the repository. Once you have a local copy of the code, follow these steps:
Open the sample project in Xcode or Finder.
Select YTPlayerView.h
, YTPlayerView.m
, and the
Assets folder. If you open the workspace in Xcode, these are available
under Pods -> Development Pods -> YouTube-Player-iOS-Helper and
Pods -> Development Pods -> YouTube-Player-iOS-Helper -> Resources. In the Finder,
these are available in the project's root directory in the Classes and
Assets directories.
Drag these files and folders into your project. Make sure the Copy items into
destination group’s folder option is checked. When dragging the Assets folder, make
sure that the Create Folder References for any added folders option is
checked.
The library should now be installed.
YTPlayerView
via Interface Builder or the Storyboard To add a YTPlayerView
via Interface Builder or the Storyboard:
Drag a UIView
instance onto your View.
Select the Identity Inspector and change the class of the view to
YTPlayerView
.
Open ViewController.h
and add the following header:
#import “YTPlayerView.h”
Also add the following property:
@property(nonatomic, strong) IBOutlet YTPlayerView *playerView;
In Interface Builder, create a connection from the View element that you defined in the
previous step to your View Controller's playerView
property.
Now open ViewController.m
and add the following code to the end of your
viewDidLoad
method:
[self.playerView loadWithVideoId:@"M7lc1UVf-VE"];
Build and run your application. When the video thumbnail loads, tap the video thumbnail to
launch the fullscreen video player.
The ViewController::loadWithVideoId:
method has a variant,
loadWithVideoId:playerVars:
, that allows developers to pass additional player
variables to the view. These player variables correspond to the
player parameters in the
IFrame Player API. The playsinline
parameter enables the video to play
directly in the view rather than playing fullscreen. When a video is playing inline, the
containing iOS application can programmatically control playback.
Replace the loadWithVideoId:
call with this code:
NSDictionary *playerVars = @{
@"playsinline" : @1,
};
[self.playerView loadWithVideoId:@"M7lc1UVf-VE" playerVars:playerVars];
Open up the storyboard or Interface Builder. Drag two buttons onto your View, labeling them
Play and Stop. Open ViewController.h
and add these methods, which
will be mapped to the buttons:
- (IBAction)playVideo:(id)sender;
- (IBAction)stopVideo:(id)sender;
Now open ViewController.m
and define these two functions:
- (IBAction)playVideo:(id)sender {
[self.playerView playVideo];
}- (IBAction)stopVideo:(id)sender {
[self.playerView stopVideo];
}
Most of the IFrame Player API functions have Objective-C equivalents, though some of the
naming may differ slightly to more closely match Objective-C coding guidelines. Notable
exceptions are methods controlling the volume of the video, since these are controlled by
the phone hardware or with built in UIView
instances designed for this purpose,
such as MPVolumeView
.
Open your storyboard or Interface Builder and control-drag to connect the Play and
Stop buttons to the playVideo:
and stopVideo:
methods.
Now build and run the application. Once the video thumbnail loads, you should be able to
play and stop the video using native controls in addition to the player controls.
It can be useful to programmatically handle playback events, such as playback state changes
and playback errors. In the JavaScript API, this is done with
event listeners.
In Objective-C,this is done with a
delegate.
The following code shows how to update the interface declaration in
ViewController.h
so the class conforms to the delegate protocol. Change
ViewController.h
’s interface declaration as follows:
@interface ViewController : UIViewController<YTPlayerViewDelegate>
YTPlayerViewDelegate
is a protocol for handling playback events in the player.
To update ViewController.m
to handle some of the events, you first need to set
the ViewController
instance as the delegate of the YTPlayerView
instance. To make this change, add the following line to the viewDidLoad
method
in ViewController.h
.
self.playerView.delegate = self;
Now add the following method to ViewController.m
:
- (void)playerView:(YTPlayerView *)playerView didChangeToState:(YTPlayerState)state {
switch (state) {
case kYTPlayerStatePlaying:
NSLog(@"Started playback");
break;
case kYTPlayerStatePaused:
NSLog(@"Paused playback");
break;
default:
break;
}
}
Build and run the application. Watch the log output in Xcode as the player state changes.
You should see updates when the video is played or stopped.
The library provides the constants that begin with the kYT*
prefix for
convenience and readability. For a full list of these constants, look at
YTPlayerView.m
.
The library builds on top of the IFrame Player API by creating a WebView
and
rendering the HTML and JavaScript required for a basic player. The library's goal is to be
as easy-to-use as possible, bundling methods that developers frequently have to write into a
package. There are a few limitations that should be noted:
YTPlayerView
instances. If your application has multipleYTPlayerView
instances, a recommended best practice is to pause or stopNSNotificationCenter
to dispatch notifications that playback is about toYTPlayerView
instances. Reuse your existing, loaded YTPlayerView
instances when possible. When aUIView
instance or aYTPlayerView
instance, and don't call either loadVideoId:
orloadPlaylistId:
. Instead, use thecueVideoById:startSeconds:
family of functions, which do notWebView
. There is a noticeable delay when loading the entireSince this is an open-source project, please submit fixes and improvements to the
master branch of the GitHub
project.
#1. YouTube-Player-iOS-Helper - GitHub
Lightweight helper library that allows iOS developers to add inline playback of YouTube videos through a WebView - GitHub ...
#2. Embed YouTube Videos in iOS Applications with the YouTube ...
The youtube-ios-player-helper is an open source library that helps you embed a YouTube iframe player into an iOS application.
#3. youtube-ios-player-helper-swift on CocoaPods.org
youtube -ios-player-helper-swift · Helper library for iOS developers looking to add YouTube video playback in their applications via the iframe player in a ...
#4. iOS API youtube-ios-player-helper 無法播放 - iT 邦幫忙
</iframe>就可以播放, 我想問的是,在用youtube-ios-player-helper下如何解決「無法播放影片」的問題?
#5. How do I use youtube-ios-player-helper in a SwiftUI project?
Since YTPlayerView is a UIView , you'll need to wrap it in UIViewRepresentable to use it in SwiftUI: import YouTubeiOSPlayerHelper struct ...
#6. youtube-ios-player-helper – opensource.google
Helper library for iOS developers looking to add YouTube video playback in their applications via the iframe player in a UIWebView ...
#7. youtube-ios-player-helper-swift - A full swift implementation of ...
A <YouTube /> component for React Native. Uses Google's official youtube-ios-player-helper for iOS and YouTube Android Player API for Android and exposes much ...
#8. Can we get normal quality of YouTube video using Safari ...
Or good quality is only possible with official YouTube app on iOS? I was trying to use the next iOS ... https://github.com/youtube/youtube-ios-player-helper.
#9. ayo1103/Test-Youtube-ios-player-helper - Giters
林昌佑| Ayo Lin Test-Youtube-ios-player-helper: 測試使用swift 串接youtube-ios-player-helper 的功能與實作.
#10. 我可以为youtube-ios-player-helper 静音吗? - IT工具网
我正在使用youtube-ios-player-helper 在我的应用程序中播放youtube 视频。我希望能够静音。这是我所做的: 在YTPlayerView 中添加setVolume() 和mute() 函数
#11. YouTube-Player-iOS-Helper - mirrors - CODE CHINA
YouTube -Player-iOS-Helper is available through CocoaPods. To install the library, add the following line to your Podfile and replace "x.y.z" ...
#12. 关于swift:具有YouTube v3 API和youtube-ios-player-helper的 ...
iOS app with YouTube v3 API and youtube-ios-player-helper can't autoplay videos我在使用Google / YouTube提供的youtube-ios-player-helper窗格 ...
#13. youtube-ios-player-helper-swift - Cocoa Controls
youtube -ios-player-helper-swift. Apache 2.0 License. Swift. iOS. Favorite ... to add YouTube video playback in their applications via the iframe player in a ...
#14. Add YouTube Video in App Swift 5 (Xcode 11) - 2020 Beginners
In this video we will learn how to add a Youtube Video into our iOS App using Swift and Xcode 11. This is ...
#15. YouTube - IOS _程式人生
我正在嘗試實現youtube-ios-player-helper,可在此處找到:https://github.com/youtube/youtube-ios-player-helper 我做了什麼:
#16. [Solved] Bug in 'YouTube iOS Player Helper' library - Code ...
I have been using the 'YouTube iOS Player Helper' library for playback of our youtube videos in our iOS App (iPhone).Today I noticed a bug in the same, ...
#17. Browse 3rd Party Forks / youtube-ios-player-helper - Public ...
To run the example project; clone the repo, and run pod install from the Project directory first. Requirements. Installation. YouTube-Player-iOS-Helper is ...
#18. Youtube-ios-player-helper Background Color - Blogger
Play Video Audio Of Video In Background Ios Issue 38 Youtube Youtube Ios Player Helper Github. Using the YouTube-Player-iOS-Helper is it possible to not ...
#19. 我該如何使用Youtube iOS API進行搜尋? - IT閱讀
我在這裡與 YouTube-Player-iOS-Helper 一起玩過:https://github.com/youtube/youtube-ios-player-helper 但是我不知道如何從某個特定的時間開始將視訊嵌入到iOS App中 ...
#20. youtube-ios-player-helper - AppSight
youtube -ios-player-helper - SDK details, apps using, related SDKs. ... Helper library for iOS developers that want to embed YouTube videos in
#21. youtube-ios-player-helper.podspec.json | searchcode
1{ 2 "name": "youtube-ios-player-helper", 3 "version": "0.1.0", 4 "summary": "Helper library for iOS developers that want to embed YouTube videos in\n their ...
#22. Youtube iOS Player Helper library didn't work - StackGuides
Unfortunately this youtube iOS helper has many issues, like layout, file locations and so on.. I have drafted a bit improved version for my own use but, ...
#23. Youtube iOS Player Helper library didn't work
I tried to implement the youtube-ios-player-helper library to play videos in my app. I can get the player ready and load the video.
#24. NicholasMata/YoutubePlayer as Swift Package - Swiftpack.co
YoutubePlayer. A port of youtube-ios-player-helper written in Swift with SPM support. Integration/Installation. Swift Package Manager (SPM) Add the ...
#25. youtube-ios-player-helper-swift 上的UIWebView 到WKWebView
我的应用程序使用框架youtube-is-player-helper-swift,在这里找到:ht.
#26. supporting of Livestreams - Youtube/Youtube-Ios-Player-Helper
Full Name, youtube/youtube-ios-player-helper. Language, Objective-C. Created Date, 2014-04-07. Updated Date, 2021-10-06. Star Count, 1407. Watcher Count, 96.
#27. Integrating YouTube into Your iOS Applications - CODE ...
Run YouTube Videos in iOS Applications. ... pod "youtube-ios-player-helper", "~> 0.1.4". Once saved, launch terminal and navigate to the ...
#28. Is youtube-ios-Player-Helper the library that plays - DDCODE
Rthttps://github.com/youtube/youtube-ios-player-helperAre there other libraries?
#29. Как правильно использовать YouTube iOS Player Helper?
Как правильно использовать YouTube iOS Player Helper? Я пытаюсь загрузить видео/плейлисты из YouTube (личный кабинет) с помощью Swift. Я добавил для этого класс ...
#30. Credits – Romeo Lab
youtube -ios-player-helper ( iOS ). Developer: google. Project code: https://github.com/youtube/youtube-ios-player-helper.
#31. YouTube-Player-iOS-Helper can't use ... - TipsForDev
The YTPlayerView is in the separate Pods module which you need to import. My Podfile: platform :ios, '8.3' target 'MyApp' do use_frameworks! pod ...
#32. youtube-ios-player-helper介绍, 评价和相关技术栈
youtube -ios-player-helper. Helper library for iOS developers that want to embed YouTube videos in their iOS apps with the iframe player API.
#33. Support For youtube-ios-player-helper - XS:CODE
Lightweight helper library that allows iOS developers to add inline playback of YouTube videos through a WebView. Services available ! ? Need anything else?
#34. YouTube-Player-iOS-Helper无法使用YTPlayerView类 - 码农 ...
我正在尝试实现YouTube iOS播放器帮助程序,可在此处找到:https://github.com/youtube/youtube-ios-player-helper我所做的:编辑了我的pod文件,pod ...
#35. 在Swift中使用YouTube-Player-iOS-Helper時找不到 ...
我遇到了與帖子YouTube-Player-iOS-Helper can't use YTPlayerView class中相同的問題。 但是,當我在後面的回答中發現https://stackoverflow.com/a/30719229在橋接頭 ...
#36. youtube-ios-player-helper - AllowMe.in
YouTube -Player-iOS-Helper is available through CocoaPods. To install the library, add the following line to your Podfile and replace "x.y.z" with the latest ...
#37. How to embed YouTube videos into your iOS app using Swift
YouTube Player iOS Helper is the official way to embed a youtube video in your iOS app. It's a recommended method if you don't want to ...
#38. 是用youtube-ios-player-helper 这个库吗? - Delphi
原本这个系统名为iPhone OS,直到2010年6月7日WWDC 大会上宣布改名为iOS。 ... RT https://github.com/youtube/youtube-ios-player-helper 还是有其他的库?
#39. Play the video of vevo using the YouTube-Player-iOS-Helper ...
Play the video of vevo using the library "YouTube-Player-iOS-Helper" for playing youtube videos on iOS. Perhaps, that could be the use of the UIWebview even ...
#40. YouTube-Player-iOS-Helper can't use YTPlayerView class
Question or problem in the Swift programming language: I am trying to implement the youtube-ios-player-helper, ...
#41. Kyle的程式學習記錄
https://github.com/youtube/youtube-ios-player-helper 安裝跟使用方式上面網址都有,這邊僅列出遇到的問題。 -- 1. 裝好之後無法使用,出現錯誤
#42. [Swift] YouTube-Player-iOS-Helper を使って YouTube 動画を ...
はじめに 先日、クラスメソッド関連会社の underscore よりマイクラTubeという、YouTube上にあるマインクラフト動画を再生するアプリをリリースしま ...
#43. youtube-ios-player-helper - Upload app to AppStore
5.2.3 Audio/Video Downloading: Apps should not facilitate illegal file sharing or include the ability to save, convert, or download media from third-party ...
#44. youtube-ios-player-helper-swift | #IOS | full swift implementation
Implement youtube-ios-player-helper-swift with how-to, Q&A, fixes, code snippets. kandi ratings - Low support, No Bugs, No Vulnerabilities.
#45. [LIB youtube-ios-player-helper] - VER 1.0.2 DONT WORKING
[LIB youtube-ios-player-helper] - VER 1.0.2 DONT WORKING. youtube. 16 July 2020 Posted by hoangduong536. HI ADMIN. VER 1.0.2 DONT WORKING, VER CURRENT: ...
#46. iOSアプリにYoutubeプレイヤーを組み込む - Qiita
youtube -ios-player-helperが使用されていますが、これは現在Apple非推奨となっているUIWebViewを使っていますので、この記事では推奨のWKWebViewに ...
#47. Play Youtube Videos in Background Using Youtube iOS ...
It is an open source library for iOS developers to help them embeding a YouTube iframe player into an iOS application or we can say that, it is a helper library ...
#48. youtube ios player helper example xmgbdyztl
go full screen, developers.google.com. so i ditched the helper, The player didn't like playing back the overly large 4K file,.
#49. youtube-ios-player-helper
Helper library for iOS developers looking to add YouTube video playback in their applications via the iframe player in a UIWebView.
#50. YouTube-Player-iOS-Helper не может использовать класс ...
Я пытаюсь реализовать youtube-ios-player-helper, который можно найти здесь: https: // github.com/youtube/youtube-ios-player-helper Что я сделал: ...
#51. import youtube-ios-player-helper (ef4d4a3f) · Commits
See the License for the specific language governing permissions and. # limitations under the License. # YouTube-Player-iOS-Helper CHANGELOG.
#52. How To Embed YouTube Videos in an iOS App with Swift 4
It seems as though the helper library youtube-ios-player-helper does not play nicely with Swift 4 or iOS 12 anymore.
#53. youtube-ios-player-helper-swift 上的UIWebView 到WKWebView
我的应用程序使用框架youtube is player helper swift,在这里找到: https : github.com malkouz youtube ios player helper swift它是原始youtube ...
#54. 是用youtube-ios-player-helper 这个库吗? - SegmentFault 思否
ios 中播放youtube视频, 是用youtube-ios-player-helper 这个库吗? HanTsing. 482. 更新于2015-10-21. RT https://github.com/youtube/youtube-ios-player-helper
#55. YouTube-Player-iOS-Helper不能使用YTPlayerView类
我正在尝试实现youtube-ios-player-helper,可在此处找到:https://gi.
#56. youtube-ios-player-helper · GitHub Topics
youtube -ios-player-helper · Here is 1 public repository matching this topic... · Improve this page · Add this topic to your repo.
#57. 我可以为youtube-ios-player-helper静音吗? - Thinbug
我正在使用youtube-ios-player-helper在我的应用程序中播放youtube视频。我希望能够静音。这是我做的:
#58. 此視頻受限制。嘗試使用Google Apps帳戶登錄。 - Thercb
youtube -ios-player-helper消息:“此視頻受限制。嘗試使用Google Apps帳戶登錄。” ... 提供的YTPlayerView在iOS應用中播放嵌入式youtube視頻。
#59. FFmpeg
AudioToolbox output device; MacCaption demuxer; PGX decoder; chromanr video filter; VDPAU accelerated HEVC 10/12bit decoding; ADPCM IMA Ubisoft APM encoder ...
#60. youtube-ios-player-helper“稍后播放按钮”不起作用
使用youtube-ios-player-helper库。很有用。但是我注意到“以后播放”按钮不适用于该库。当它被按下时,它变成一个“!”。它从.
#61. Adobe Flash Player - Wikipedia
AIR supports installable applications on Windows, Linux, macOS, and some mobile operating systems such as iOS and Android. Flash applications must specifically ...
#62. Mobalytics - The All-in-One Companion for Every Gamer
The all-in-one gaming companion that analyzes your performance and gives you insights to level up your game.
#63. How to Download YouTube Videos | PCMag
Pick a video in the library for quick conversion to MP4, MP3, or even ACC (the audio format preferred by iOS devices). The player didn't like playing back the ...
#64. My Playbook | Fantasy Football | FantasyPros
Identify the player that have favorable matchups. Learn More. Player News & Notes. Stay on top of the latest player information.
#65. oTranscribe
Private - your audio file and transcript never leave your computer. Export to Markdown, plain text and Google Docs. Video file support with integrated player.
#66. Brawl Stars Support
I didn't get my iOS purchase! Payment methods on Android. Logo. Gameplay. How to Play. How to get ...
#67. iOS app with YouTube v3 API and youtube-ios-player-helper ...
I am having a problem autoplaying videos with the youtube-ios-player-helper pod provided by Google/YouTube. Here is the relevant part of my ...
#68. Youtube使用youtube-ios-player-helper在iOS 11中播放没有 ...
swift4中Youtube使用youtube-ios-player-helper在iOS 11中播放没有音频的视频,我正在尝试使用youtube-ios-player-helper集成Youtube视频这是在iOS ...
#69. Material Design Accessibility
Build beautiful, usable products faster. Material Design is an adaptable system—backed by open-source code—that helps teams build high quality digital ...
#70. 連結裝置以便在電視上看YouTube - iPhone 和iPad
確認iPhone 或iPad 已連上智慧型電視或串流裝置使用的Wi-Fi 網路。 開啟YouTube 應用程式。 選取要觀看的影片,然後輕觸「投放」圖示 。 如果裝置是iOS ...
#71. Psyonix Support - Rocket League
Welcome to Rocket League Support! This is the best place to get all your questions answered and your issues resolved. You can search for our Help Center for ...
#72. Buy Plugins & Code from CodeCanyon
Discover 23371 Plugins, Code and Script for Bootstrap, Javascript, PHP, Wordpress, HTML5 and more. Save time, buy Code on CodeCanyon!
#73. TripIt - Highest-rated trip planner and flight tracker
Instagram Facebook Twitter Linkedin Youtube Tripit Blog · TripIt -. Visit TripIt's new Traveler Resource Center—Your source for travel policies, ...
#74. Joycon droid apk reddit - Johnsrud Transport
App Description. For many, a smartphone is a music player that just happens to have a built-in communication device. Phone support: iOS, Android Connectivity: ...
#75. Dreame mod apk latest version - Les Tontons
Follow these few steps to Install Avee Player Pro + Mod APK: Download the ... Dreame cheat engine without human verification android ios Mobile #chrome #pc ...
#76. Spotify api wordpress
The audio player works on iPhone, iPad, Android, Firefox, Chrome, Safari, Opera, Internet Explorer 11 and Microsoft Edge. Cosmic based music blog with ...
#77. Youtube downloader apk 2019
This YouTube Go Android & iOS/iPhone is available for Untuk PC, ... Oct 21, 2021 · YouTube for Android is a player app specially designed to be ...
#78. Beatstar ios hack
All-in-One iOS Helper Brings Useful, Delightful Features Jul 06, ... Toca y desliza el dedo hacia los instrumentos Youtube Vanced on iOS & ...
#79. Samsung music apkmirror - Location voiture Tunisie
MediaMonkey is a bit of a dark horse in the music player apps business. KingoRoot is developed in apk file format. APKMirror Installer is a helper app that ...
#80. Fb story to mp4
Why would someone want to save video from Youtube (or Facebook for that ... It is possible to open MP4 file with almost any player on Windows but on Mac you ...
#81. Blooket hack coins
The new developer is Adam TschanTheFinalWillow. io helper JS - Remember words Author ... How to make unlimited coins in Blooket - YouTube › Search www.
#82. Why/how is YouTube blocking all the native iOS video player ...
Why/how is YouTube blocking all the native iOS video player controls in embedded YouTube vids? You don't have the option to full screen, ...
#83. Deezer music download
Deezer on Android ; Deezer on iOS ; Deezer on Windows Mobile ; Deezer on ... to be a good helper to achieve these goals by downloading Spotify songs to MP3.
#84. Ue4 retainer box
61030 Epic Games Launcher GameInput Redistributable Google Update Helper ... allows the player to record an answer in voice, or create a new ask-all.
#85. Yandex video downloader online - Risata
Next, go to JW Player and look for the video that you want to download. ... YouTube, Facebook, Vimeo, Vevo, Dailymotion, Hulu, and adult sites are supported ...
#86. Gbf Discord Bot
Thanks to fixitfixit discord and YouTube. Glacial Augment is the third and final page that I ... They can give a player a variety of RNG-based rewards.
#87. TubeBuddy | The Premier YouTube Channel Management ...
Your best friend on the road to YouTube success. TubeBuddy is a FREE browser extension & mobile app that integrates directly into YouTube to help you run ...
#88. Vr player windows - Poker Online
GoPro VR Player is available to all software users as a free download for Windows/5(40). ... It supports playing VR videos on Windows, iOS, and Android.
#89. Daily Fantasy Basketball | FantasyLabs
Get daily fantasy basketball analysis, news and tools to help you build smarter DFS lineups for DraftKings and Fanduel.
#90. Mod App - Radio Reekenfeld
WeMod is the world's best application for modding hundreds of single-player PC games. May 18, 2021 · Tutu helper app for android, ios and pc.
#91. The Top 5 Apps for Hosting a Secret Santa Gift Exchange
We've rounded up the best Secret Santa apps for Android and iOS to help ... Secret Santas Helper is another nice app to get to grips with, ...
#92. YouTube on iOS - Aux Mode
Touch the. menu icon in the video player to access additional viewing options. ... Make sure you have the latest version of the YouTube iOS app installed.
#93. Download Youtube++ with no ads for free on iOS - Panda ...
For those want to remove the ads in Youtube++, we have a method for you. Youtube++ with no ads is available on Panda Helper.
#94. Blue Jackets Sunday Gathering: Jack Roslovic has yet to join ...
But there's one player who has yet to join the party: center Jack ... Voracek had the primary helper on the first NHL goals of Oskar ...
youtube-ios-player-helper 在 YouTube-Player-iOS-Helper - GitHub 的推薦與評價
Lightweight helper library that allows iOS developers to add inline playback of YouTube videos through a WebView - GitHub ... ... <看更多>
相關內容